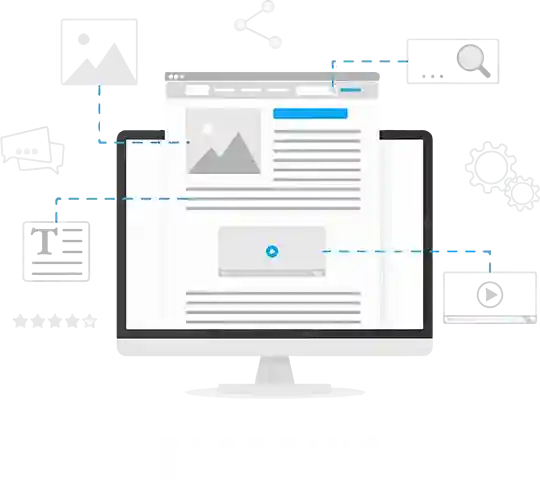
Hemanshu Kalsariya - September 21, 2021
A modal is a pop-up dialogue that appears on top of the app's content and must be closed before interaction may proceed.
It can be used as a select component when there are many options to choose from or to filter items in a list, among other things.
The dismiss() method on the modal controller can be used to dismiss the modal once it has been created.
After the modal has been dismissed, the onDidDismiss function can be used to do an action.
Using scoped encapsulation, Modal will automatically scope its CSS at runtime by adding an extra class to each of the styles.
In CSS, overriding scoped selectors necessitates a selector with higher specificity.
We recommend utilizing the create method to pass a custom class to cssClass and using it to add custom styles to the host and inner elements.
Multiple classes can be separated by spaces in this property.
To understand how to pass a class using cssClass, you can refer the Usage section.
.modal-wrapper { background: #222; }
This CSS class does not work.
.my-custom-class .modal-wrapper { background: #222; }
Works pass “my-custom-class” in cssClass to increase specificity.
Without the need of targeting specific elements, any of the provided CSS Custom Properties can be used to customize the Modal:
.my-custom-class { --background: #222; }
import { Component } from '@angular/core'; import { ModalController } from '@ionic/angular'; import { ModalPage } from '../modal/modal.page'; @Component({ selector: 'modal-page', templateUrl: 'modal-page.html', styleUrls: ['./modal-page.css'] }) export class ModalExample { constructor(public modalController: ModalController) { } async presentModal() { const modal = await this.modalController.create({ component: ModalPage, cssClass: 'my-customclass' }); return await modal.present(); } }
import { Component, Input } from '@angular/core'; @Component({ selector: 'modal-page', }) export class ModalPage { constructor() {} }
Use a (div class="ion-page") as a wrapper element inside your modal component so that the component dimensions are still calculated correctly.
Data can be provided via componentProps during the establishment of a modal. The following data can be added to the preceding example
async presentModal() { const modal = await this.modalController.create({ component: ModalPage, cssClass: 'my-customclass', componentProps: { 'firstName': 'Douglas', 'lastName': 'Adams', 'middleInitial': 'N' } }); return await modal.present(); }
To passed data into the componentProps, set it as an @Input:
export class ModalPage { // Data passed in by componentProps @Input() firstName: string; @Input() lastName: string; @Input() middleInitial: string; }
By invoking the dismiss method on the modal controller and optionally supplying any data from the modal, a modal can be dismissed.
export class ModalPage { ... dismiss() { this.modalController.dismiss({ 'dismissed': true }); } }
The data can be read in through the onWillDismiss or onDidDismiss linked to the modal after it has been dismissed:
const { data } = await modal.onWillDismiss(); console.log(data);
When lazy loading a modal, remember that the modal will be loaded when the module (that imports the modal's module) is loaded, not when the modal is opened.
Consider the case of a CalendarComponent and an EventModal.
By clicking a button in the CalendarComponent, the modal appears.
Because the modal is produced in the CalendarComponentModule, the EventModalModule must be included in the CalendarComponentModule in Angular:
import { NgModule } from '@angular/core'; import { CommonModule } from '@angular/common'; import { IonicModule } from '@ionic/angular'; import { CalendarComponent } from './calendar.component'; import { EventModalModule } from '../modals/event/event.module'; @NgModule({ declarations: [ CalendarComponent ], imports: [ IonicModule, CommonModule, EventModalModule ], exports: [ CalendarComponent ] }) export class CalendarComponentModule {}
Modals in iOS mode can be presented in a card-like format and swiped to close.
The card-style display and the swipe to close action aren't mutually exclusive, so you can employ whichever features you like.
On iPhone-sized devices, card-style models do not have a background. As a result, the --background-opacity parameter has no impact.
If you are building an application that makes use of ion-tabs, it is suggested to bring up ion-router-outlet using this.routerOutlet.parentOutlet.nativeEl, or else when the modal opens, the tab bar will not scale down.
import { IonRouterOutlet } from '@ionic/angular'; constructor(private routerOutlet: IonRouterOutlet) {} async presentModal() { const modal = await this.modalController.create({ component: ModalPage, cssClass: 'my-customclass', swipeToClose: true, presentingElement: this.routerOutlet.nativeEl }); return await modal.present(); }
Using the ion-router-outlet element as the presentingElement is fine in most cases.
The top-most ion-modal element should be utilized as the presentingElement when showing a card-style modal from within another modal.
import { ModalController } from '@ionic/angular'; constructor(private modalController: ModalController) {} async presentModal() { const modal = await this.modalController.create({ component: ModalPage, cssClass: 'my-customclass', swipeToClose: true, presentingElement: await this.modalController.getTop() }); return await modal.present(); }
Name | Description |
---|---|
ionModalDidDismiss | After the modal has been discarded, this is emitted. |
ionModalDidPresent | After the modal has been provided, this is emitted. |
ionModalWillDismiss | Before the modal has been discarded, this is emitted. |
ionModalWillPresent | Before the modal has appeared, this is emitted. |
After the modal overlay has been provided, dismiss it.
Signature:
dismiss(data?: any, role?: string | undefined) => Promise2) onDidDismiss():
When the modal was dismissed, this method returns a promise that resolves.
Signature:
onDidDismiss3) onWillDismiss():() => Promise >
Returns a promise indicating when the modal will be dismissed.
Signature:
4) Present():onWillDismiss () => Promise >
After the modal overlay has been generated, present it.
Signature:
present() => Promise
Name | Description |
---|---|
---backdrop-opacity | The opacity of the backdrop |
--background | Background of the model content |
--border-color | Border color of the model content |
--border-radius | Border radius of the model content |
--border-style | Border style of the model content |
--border-width | The border width of the model content |
--height | Height of the model |
--max-height | Maximum height of the model |
--min-height | Minimum height of the model |
--max-width | Maximum width of the model |
--min-width | Minimum width of the model |
--width | Width of model |
In this blog, we have seen what is ion-model in the Ionic framework and how to use ion-model in your project. As we see above We can use ion-model as a select component when there are many options to choose from or to filter items in a list.
January 23, 2023
September 21, 2021
September 20, 2021
July 23, 2021
July 06, 2021
Technology that meets your business requirements
Planning a cutting-edge technology software solution? Our team can assist you to handle any technology challenge. Our custom software development team has great expertise in most emerging technologies. At iFour, our major concern is towards driving acute flexibility in your custom software development. For the entire software development life-cycle, we implement any type of workflow requested by the client. We also provide a set of distinct flexible engagement models for our clients to select the most optimal solution for their business. We assist our customers to get the required edge that is needed to take their present business to next level by delivering various out-reaching technologies.
Get advanced technology that will help you find the
right answers for your every business need.
Get in touch
Drop us a line! We are here to answer your questions 24/7.