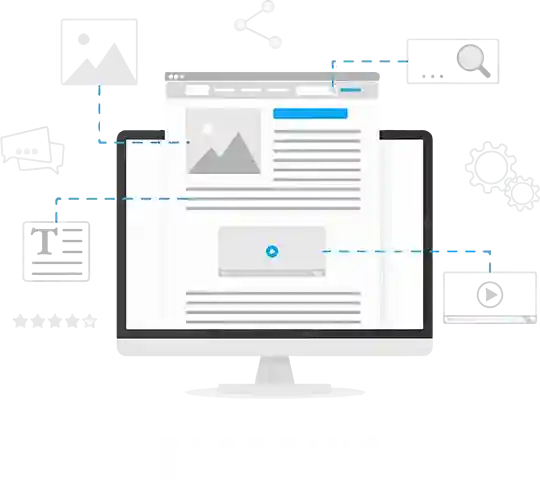
Hemangi Tank - September 20, 2021
It is a general control that is used to display multiple items in a drop-down format. It is called ComboBox because it is a combination of Textbox and a drop-down list. In Textbox, we can write the content which we want to find or add items.
In ComboBox, only a single item will be seen instead of the whole collection of items as in the List box. Also, only a single item will be selected at a time in this control. Unlike the List box, in ComboBox initially no items are visible, we have to click the ComboBox and then we can see all the items present in it.
We can create, format, and present our ComboBox in multiple techniques. Some of them are:
The ComboBox control represents a different number of items in XAML. It has multiple properties available to display the ComboBox more attractively and to make it easily accessible by the user. Some of the properties are Height, Width, Background, Name, SelectedValue, Selected Index, etc.
To create ComboBox use the following code:
SimpleComboBox.xaml:
SimpleComboBox.xaml.cs:
private void btn_Click(object sender, RoutedEventArgs e) { MessageBox.Show(cmb1.Text); }
Here, we can see that we add the ComboBox items using the ComboBoxItem tag at design time and the item is visible in the output as follows.
Image: Simple ComboBox
In the above method, we saw how an item can be added at design time. Now, we will learn how to add and remove items from the ComboBox during the execution.
For this, we have to add one ComboBox, a textbox, and two buttons for the addition and deletion of ComboBox items respectively. And for this, we internally have the Add and Remove/RemoveAt methods present in the control itself.
As we have two different buttons for adding and deleting the items from the ComboBox, we can do code on their Click event handler.
In the Add button click event, we insert the content that the user entered in the textbox to the ComboBox using the Add method of ComboBox, and similarly, on the click event of the Delete button, we remove the selected item from the ComboBox using RemoveAt method of ComboBox.
Below is the design and the back-end code for this functionality.
Add-Dlt_Items.xaml:
Add-Dlt_Items.xaml.cs:
private void btn_add_Click(object sender, RoutedEventArgs e) { cmb.Items.Add(txtbox.Text); MessageBox.Show("Item Added"); } private void btn_dlt_Click(object sender, RoutedEventArgs e) { cmb.Items.RemoveAt(cmb.Items.IndexOf(cmb.SelectedItem)); }
For styling our ComboBox, we have multiple properties available such as foreground, background, fontfamily, fontsize, etc. for specific ComboBoxItems.
Here, to style and format the ComboBox we do not have to do any coding in the back-end of the file.
So, in this example, we added one ComboBox and some ComboboxItems and in all of them, we added the Background property which sets the background color of each individual ComboboxItems.
Also, in one ComboBoxItem, we have added the Image tag to add some pictures to make it good-looking and understandable at the first sight.
FormattedComboBox.xaml:
Image: Formatting of ComboBox
In this method, we can also add some checkboxes to each ComboBoxItem as we added the image in it. To do so, we can add the Stack Panel inside the Checkbox control, and then the CheckBox will be visible. And similarly, if we add CheckBox to all the ComboBoxItems then each item will be having checkbox before it.
And after this code, the output will be:
In this method, we illustrate that we can create a ComboBox dynamically also. For this, there is no need to do anything in the design part.
So, in the back-end of the file, we create one ComboBox and provides its necessary properties to it like name, width, height, etc., and then we can add items to it.
Here, we can add items in two different ways. One, creating the instance of ComboBoxItem and then adding that instance to the ComboBox, and the second is to directly add the item using Add method of the ComboBox instance itself.
The second method is easy as well as efficient as it takes less coding and the work is done effortlessly.
DynamicComboBox.xaml:
DynamicComboBox.xaml.cs:
public partial class DynamicComboBox : Window { public DynamicComboBox() { InitializeComponent(); ComboBox comboBox = new ComboBox(); comboBox.Name = "ComboBox1"; comboBox.Width = 194; comboBox.Height = 30; comboBox.IsEditable = true; ComboBoxItem item = new ComboBoxItem(); item.Content = "Coffee"; comboBox.Items.Add(item); comboBox.Items.Add("Tea"); comboBox.Items.Add("Orange"); comboBox.Items.Add("Milk"); comboBox.Items.Add("Iced Tea"); comboBox.Items.Add("Mango Shake"); window.Content = comboBox; window.Background = Brushes.Moccasin; } }
Image: Add Items Dynamically
In WPF ComboBox, we can also display data i.e. items by binding the ComboBox with an array, list, and all.
For this, in this example, we create one ComboBox in the design part and an Array at the back-end. Then, we give the reference of that array to the ItemsSource property of the ComboBox using its name.
ComboBoxBinding.xaml:
ComboBoxBinding.xaml.cs:
public partial class ComboBoxBinding : Window { public ComboBoxBinding() { InitializeComponent(); string[] str = new string[] { "AryaBhata", "A.P.J. Abdul Kalam", "C.V. Raman", "Vikram Sarabhai", "Srinivasa Ramanujan" }; cmb.ItemsSource = str; cmb.SelectedIndex = 0; } }
Image: Binding ComboBox
In this article, we saw how to create, use and format ComboBox in WPF using XAML and CS. Also, we saw how we can add, delete and alter any ComboBoxItems. In the real world, ComboBox is used when we need the user to select one item from multiple items, for example, Country, State, or any specific entry.
January 23, 2023
September 21, 2021
September 20, 2021
July 23, 2021
July 06, 2021
Technology that meets your business requirements
Planning a cutting-edge technology software solution? Our team can assist you to handle any technology challenge. Our custom software development team has great expertise in most emerging technologies. At iFour, our major concern is towards driving acute flexibility in your custom software development. For the entire software development life-cycle, we implement any type of workflow requested by the client. We also provide a set of distinct flexible engagement models for our clients to select the most optimal solution for their business. We assist our customers to get the required edge that is needed to take their present business to next level by delivering various out-reaching technologies.
Get advanced technology that will help you find the
right answers for your every business need.
Get in touch
Drop us a line! We are here to answer your questions 24/7.