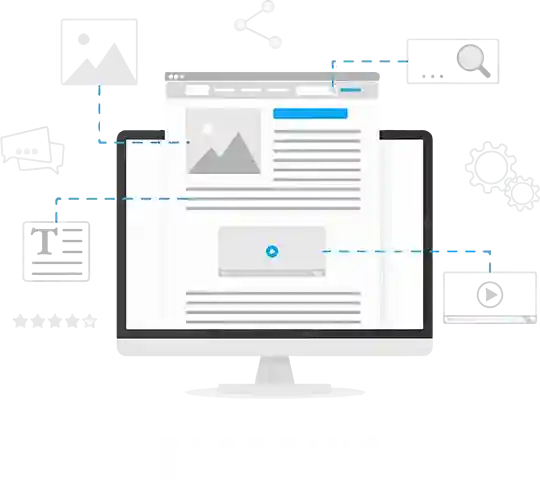
iFour Team - June 21, 2021
To use the command interface, you can specify a data binding that targets the Command property of Button where the source is a property in the ICommand type ViewModel. The ViewModel contains code related to the ICommand property that is implemented when the button is clicked. You can set CommandParameter to arbitrary data for the difference between multiple buttons if they are bound to the same ICommand property in all ViewModel.
Sometimes the View needs to include buttons that trigger various actions in the ViewModel. But the ViewModel should not make handlers Clicked for the buttons as it will link the ViewModel to an instance of a particular user interface.
Command interface uses it. This command interface is supported by the below mention elements in Xamarin.Forms:
Button
MenuItem
ToolbarItem
SearchBar
TextCell (and hence also ImageCell)
ListView
TapGestureRecognizer
All of these commands can be handled in a ViewModel in a way that does not depend on the specific user-interface object in the view.
To support the command, two public properties are defined based on the majority of this class:
Command of type System.Windows.Input.ICommand
CommandParameter of type object
To use the command interface, your ViewModel has ICommand type properties:
Public ICommand MyCommand { private set ; get ; }Implementing Command:
To implement the command, the ViewModel must define one or more properties of the ICommand type. The ICommand interface defines one event and two methods:
public interface ICommand { public void Execute (Object parameter); public bool CanExecute (Object parameter); public event EventHandler CanExecuteChanged; }
CanExecuteChanged event: See CanExecute understand a little more, but binding this command will indicate the view if the ability to execute the command has changed (value is Boolean, so true to false or false to true)
CanExecute method: If the binding element of the command defines whether the command executes. Depending on the element, this can disable the element if the method returns incorrectly. For example, a button could be grayed out, disabled, so the user does not press it. Until something changes, by enabling the CanExecute button again, true can be returned.
Execute method: Executes a function, here is where we need to call the add button from the ViewModel, in the case of this special command.
The Command and Command classes are provided by Xamarin Forms implement the ICommand interface, where T is the kind of the arguments to Execute and CanExecute. also as implement the ICommand interface, these classes include the ChangeCanExecute method, which occurs if the CanExecuteChanged event is canceled.
Within a ViewModel, there should be an object of type Command or Command for every property in the ViewModel of type ICommand. The Command or Command constructor requires an Action callback object, which is named when the Button calls the ICommand.Execute method. The CanExecute method is an alternative constructor parameter and takes the form of a function that returns a bool.
The ViewModel should also refer to a class that implements the Command interface. This class will be described shortly.
In view, Button's command property is tied to that property:
When the user presses the button, the button calls the executed method in the ICommand object tied to its command property. It is the easiest part of the commanding interface.
The CanExecute method is more complex. When the binding is first defined on Command property of the Button, and when the data binding changes in some way, the Button calls the CanExecute method in the ICommand object. If the CanExecute comes back wrong, the button can disable itself. This means that the actual command is not currently available or invalid.
The button also connects the handler to the ICommand CanExecuteChanged event. This event is deleted from within the ViewModel. When that event is fired, the button calls the CanExecute again. The button enables itself if the CanExecute comes back right and disables itself if the CanExecute gives back the wrong. When your ViewModel defines an ICommand type property, the ViewModel must also have or reference a category that applies to the ICommand interface.
This class should have or refer to executed and can exit methods, and the CanExecutevent event must be fired whenever the CanExecute method can return a different value.
You can use a category that somebody else has written. Because ICommand is an element of Microsoft Windows, it's been used for years with Windows MVVM applications. employing a Windows class that implements ICommand allows you to share your ViewModels between Windows applications and Xamarin.Forms applications.
If sharing ViewModels between Windows and Xamarin.Forms don't seem to be a priority, then you'll be able to use the Command or Command class included in Xamarin.Forms to implement the ICommand interface. These classes allow you to specify the bodies of the Execute and CanExecute methods at school constructors. Use Command once you use the CommandParameter property to differentiate between multiple views sure to the identical ICommand property, and also the simpler Command class when that won't a requirement.
Using Command ParametersThe ICommand.executed method are going to be passed to quick the command using command class. The IncreaseCommand is data-bound to the Command property of a Button.
Now create MainPage.xaml file. Create Button and Label with command property.
MainPage.xamlNow add BindingContext in the code-behind file.
MainPage.xaml.csusing System; using System.Collections.Generic; using System.ComponentModel; using System.Linq; using System.Text; using System.Threading.Tasks; using Xamarin.Forms; namespace CommandParameter { public partial class MainPage : ContentPage { public MainViewModel VM => ((MainViewModel)BindingContext); public MainPage() { InitializeComponent(); VM.IncreaseCommand.Execute("2"); } } }
Now we create INotifPropertyChanged in a separate file named MainViewmodel.cs .In this file, we can create an interface command and add IncreaseCount() method to increase the count. And also display count.
MainViewModel.csusing System; using System.Collections.Generic; using System.ComponentModel; using System.Runtime.CompilerServices; using System.Text; using System.Windows.Input; using Xamarin.Forms; namespace CommandParameter { public class MainViewModel : INotifyPropertyChanged { public MainViewModel() { IncreaseCommand = new Command(IncreaseCount); } public Command IncreaseCommand { private set; get; } int Count = 0; void IncreaseCount(string i) { if (int.TryParse(i, out int int2)) { Count +=int2; OnPropertyChanged(nameof(DisplayCount)); } } public string DisplayCount => $"You Clicked {Count} time(s)."; public event PropertyChangedEventHandler PropertyChanged; void OnPropertyChanged([CallerMemberName] string propertyName=null) { PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName)); } } }
(After click the button)
In this blog, we have learned about Interface Command in Xamarin forms. We have created a Button and Label with the command property. Then created a ViewModel and a command interface in this ViewModel. Also we have added IncreaseCount( ) method. Thus, when Button is clicked, in label, display count is increase with parameter 2.
January 23, 2023
September 21, 2021
September 20, 2021
July 23, 2021
July 06, 2021
Technology that meets your business requirements
Planning a cutting-edge technology software solution? Our team can assist you to handle any technology challenge. Our custom software development team has great expertise in most emerging technologies. At iFour, our major concern is towards driving acute flexibility in your custom software development. For the entire software development life-cycle, we implement any type of workflow requested by the client. We also provide a set of distinct flexible engagement models for our clients to select the most optimal solution for their business. We assist our customers to get the required edge that is needed to take their present business to next level by delivering various out-reaching technologies.
Get advanced technology that will help you find the
right answers for your every business need.
Get in touch
Drop us a line! We are here to answer your questions 24/7.