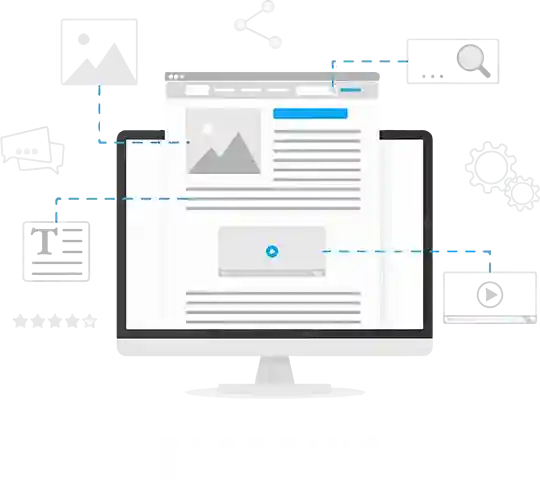
iFour Team - April 05, 2021
In this blog, we will be going to discuss how to use application indexing and Deep Linking using Xamarin.Forms. Xamarin is a cross-platform language of the .NET framework. In this language, you have to create or making mobile applications. If you have created a design in Xamarin then you have to use XAML (Extensible Application Markup Language) page for creating the design.
App indexing allows apps that will be forgotten after a few uses, appearing in search results, remaining relevant. Deep linking allows applications to respond to a search result containing application data, usually by navigating to a page referenced from a deep link. This example shows how to use app indexing and deep linking to make Xamarin.Forms app content searchable on iOS and Android devices.
Example of Deep LinkingTake an email client as an example. When the user clicks on the notification of an email they have received, they open a deep link that takes them to the email in the app. Finally, deep links also allow Google to index your app and link to specific parts of your app in searches. The deep link appears as a search result on Google and can take the user to a specific part of your app.
Please follow these steps, to implement Deep Linking in the project using SQLite Database in Xamarin.Forms.
Step 1: First you have to create a new Xamarin project for Data Linking.
1. First select the cross-platform from the left side menu.
2. Then select Mobile-app (Xamarin.Forms).
3. Then select the destination to put the project in location.
4. After declaring the unique name of your project and then click ok.
Figure 1: Create a new Xamarin Project.
5. Select the blank template.
6. Selecting your platform depending on your requirements.
7. And then press ok.
Figure 2: Select your template and Platform.
Step 2: First, Install all the packages in the project. Define all the package names below.
1)SQLitePCL_bundle_green
2)SQLitePCL_raw
3)SQLitePCL_Raw.bundle.green
4)SQLiteRaw.Core
5)SQLite-net-PCL
Figure 3: Packages Name.
Step 3: Now create three New Folders and assign the name View, ViewModel, and Model.
Step 4: Crete a Class in the Model folder and assign the name as DataItem.cs
File Name: DataItem.cs
Code:
using SQLite; namespace DeepLinking { public class DataItem { [PrimaryKey, AutoIncrement] public int ID { get; set; } public string Name { get; set; } public string Comments { get; set; } public bool Submit { get; set; } } }
Step 5: Create a new folder and assign the name as Data then create a class name as DataItemDatabase.cs
File Name: DataItemDatabase.cs
Code:
using System.Collections.Generic; using System.Threading.Tasks; using SQLite; namespace DeepLinking { public class DataItemDatabase { readonly SQLiteAsyncConnection database; public DataItemDatabase(string dbPath) { database = new SQLiteAsyncConnection(dbPath); database.CreateTableAsync().Wait(); } public Task > GetItemsAsync() { return database.Table
().ToListAsync(); } public Task > GetItemsNotDoneAsync() { return database.QueryAsync
("SELECT * FROM [DataItem] WHERE [Done] = 0"); } public Task GetItemAsync(int id) { return database.Table ().Where(i => i.ID == id).FirstOrDefaultAsync(); } public Task SaveItemAsync(DataItem item) { if (item.ID != 0) { return database.UpdateAsync(item); } else { return database.InsertAsync(item); } } public Task DeleteItemAsync(DataItem item) { return database.DeleteAsync(item); } } }
Step 6: Now create a View page in the view folder and assign the name like as DataItemPage.Xaml
File Name: DataItemPage.xaml
Code:
File Name: DataItemPage.xaml.cs
Code:
using System; using Xamarin.Forms; namespace DeepLinking { public partial class DataItemPage : ContentPage { IAppLinkEntry appLink; bool isNewItem; public DataItemPage() : this(false) { } public DataItemPage(bool isNew = false) { InitializeComponent(); isNewItem = isNew; } protected override void OnAppearing() { appLink = GetAppLink(BindingContext as DataItem); if (appLink != null) { appLink.IsLinkActive = true; } } protected override void OnDisappearing() { if (appLink != null) { appLink.IsLinkActive = false; } } async void OnSaveClicked(object sender, EventArgs e) { var todoItem = (DataItem)BindingContext; await App.Database.SaveItemAsync(todoItem); appLink = GetAppLink(BindingContext as DataItem); Application.Current.AppLinks.RegisterLink(appLink); await Navigation.PopAsync(); } async void OnDeleteClicked(object sender, EventArgs e) { var dataItem = (DataItem)BindingContext; await App.Database.DeleteItemAsync(dataItem); Application.Current.AppLinks.DeregisterLink(appLink); await Navigation.PopAsync(); } async void OnCancelClicked(object sender, EventArgs e) { await Navigation.PopAsync(); } AppLinkEntry GetAppLink(DataItem item) { var pageType = GetType().ToString(); var pageLink = new AppLinkEntry { Title = item.Name, Description = item.Comments, AppLinkUri = new Uri($"http://{App.AppName}/{pageType}?id={item.ID}", UriKind.RelativeOrAbsolute), IsLinkActive = true, Thumbnail = ImageSource.FromFile("monkey.png") }; pageLink.KeyValues.Add("contentType", "TodoItemPage"); pageLink.KeyValues.Add("appName", App.AppName); pageLink.KeyValues.Add("companyName", "Xamarin"); return pageLink; } } }
Step 7: Create a new class in the ViewModel folder and assign the name like as DataItemPage.cs.
File Name: DataItemPage.xaml.cs
Code:
using System; using Xamarin.Forms; namespace DeepLinking { public class TodoItemPageCS : ContentPage { IAppLinkEntry appLink; bool isNewItem; public TodoItemPageCS() : this(false) { } public TodoItemPageCS(bool isNew = false) { isNewItem = isNew; var datanameEntry = new Entry { Placeholder = "task name" }; datanameEntry.SetBinding(Entry.TextProperty, "Name"); var commentEntry = new Entry(); commentEntry.SetBinding(Entry.TextProperty, "Comments"); var SubmitSwitch = new Switch(); SubmitSwitch.SetBinding(Switch.IsToggledProperty, "Submit"); var saveButton = new Button { Text = "Save" }; saveButton.Clicked += OnSaveClicked; var removeButton = new Button { Text = "remove" }; removeButton.Clicked += OnDeleteClicked; var cancelButton = new Button { Text = "Cancel" }; cancelButton.Clicked += OnCancelClicked; Title = "Todo Item"; Content = new StackLayout { Margin = new Thickness(20), VerticalOptions = LayoutOptions.StartAndExpand, Children = { new Label { Text = "Name" }, datanameEntry, new Label { Text = "Comments" }, commentEntry, new Label { Text = "Submit" }, SubmitSwitch, saveButton, deleteButton, cancelButton } }; } protected override void OnAppearing() { appLink = GetAppLink(BindingContext as DataItem); if (appLink != null) { appLink.IsLinkActive = true; } } protected override void OnDisappearing() { if (appLink != null) { appLink.IsLinkActive = false; } } async void OnSaveClicked(object sender, EventArgs e) { var todoItem = (DataItem)BindingContext; await App.Database.SaveItemAsync(todoItem); appLink = GetAppLink(BindingContext as DataItem); Application.Current.AppLinks.RegisterLink(appLink); await Navigation.PopAsync(); } async void OnDeleteClicked(object sender, EventArgs e) { var todoItem = (DataItem)BindingContext; await App.Database.DeleteItemAsync(todoItem); Application.Current.AppLinks.DeregisterLink(appLink); await Navigation.PopAsync(); } async void OnCancelClicked(object sender, EventArgs e) { await Navigation.PopAsync(); } AppLinkEntry GetAppLink(DataItem item) { var pageType = GetType().ToString(); var pageLink = new AppLinkEntry { Title = item.Name, Description = item.Comments, AppLinkUri = new Uri($"http://{App.AppName}/{pageType}?id={item.ID}", UriKind.RelativeOrAbsolute), IsLinkActive = true, Thumbnail = ImageSource.FromFile("monkey.png") }; pageLink.KeyValues.Add("contentType", "DataItemPage"); pageLink.KeyValues.Add("appName", App.AppName); pageLink.KeyValues.Add("companyName", "Xamarin"); return pageLink; } } }
Step 8: Create a new view page for showing the list of data for searching the app using Goggle API.
File Name: DataListPage.xaml
Code:
File Name: DataListItem.xaml.cs
Code:
using System; using Xamarin.Forms; namespace DeepLinking { public partial class DataListItemPage : ContentPage { public DataListItemPage() { InitializeComponent(); } protected override async void OnAppearing() { base.OnAppearing(); listView.ItemsSource = await App.Database.GetItemsAsync(); } protected override void OnDisappearing() { base.OnDisappearing(); listView.ItemsSource = null; } async void OnAddItemClicked(object sender, EventArgs e) { await Navigation.PushAsync(new DataItemPage { BindingContext = new DataItem() }); } async void OnItemSelected(object sender, SelectedItemChangedEventArgs e) { if (e.SelectedItem != null) { await Navigation.PushAsync(new DataItemPage { BindingContext = e.SelectedItem as DataItem }); } } } }
Step 9: Show all the data in the listview at that time you have all the data fetch using API and add in listview.
File Name: DataListPage.cs
Code:
using System; using Xamarin.Forms; namespace DeepLinking { public partial class DataListPageCS : ContentPage { ListView listView; public DataListPageCS() { var toolbarItem = new ToolbarItem { Text = "+" }; toolbarItem.Clicked += OnAddItemClicked; ToolbarItems.Add(toolbarItem); var dataTemplate = new DataTemplate(() => { var label = new Label { HorizontalOptions = LayoutOptions.StartAndExpand, VerticalTextAlignment = TextAlignment.Center }; label.SetBinding(Label.TextProperty, "Name"); var image = new Image { Source = ImageSource.FromFile("thumb.png"), HorizontalOptions = LayoutOptions.End }; image.SetBinding(VisualElement.IsVisibleProperty, "Submit"); var stackLayout = new StackLayout { Orientation = StackOrientation.Horizontal, Children = { label, image } }; return new ViewCell { View = stackLayout }; }); listView = new ListView { ItemTemplate = dataTemplate, Margin = new Thickness(20) }; listView.ItemSelected += OnItemSelected; Title = "Data"; Content = listView; } protected override async void OnAppearing() { base.OnAppearing(); listView.ItemsSource = await App.Database.GetItemsAsync(); } protected override void OnDisappearing() { base.OnDisappearing(); listView.ItemsSource = null; } async void OnAddItemClicked(object sender, EventArgs e) { await Navigation.PushAsync(new DataItemPage { BindingContext = new DataItem() }); } async void OnItemSelected(object sender, SelectedItemChangedEventArgs e) { if (e.SelectedItem != null) { await Navigation.PushAsync(new DataItemPage { BindingContext = e.SelectedItem as DataItem }); } } } }
In this blog, we have explained how to use application indexing and Deep Linking using Xamarin.Forms.App Indexing involves indexing your app on Google Search and Apple's Mobile Search for a user to find. Deep linking involves a user clicking a search result and taking it to the correct page and viewing the correct content in your app.
January 23, 2023
September 21, 2021
September 20, 2021
July 23, 2021
July 06, 2021
Technology that meets your business requirements
Planning a cutting-edge technology software solution? Our team can assist you to handle any technology challenge. Our custom software development team has great expertise in most emerging technologies. At iFour, our major concern is towards driving acute flexibility in your custom software development. For the entire software development life-cycle, we implement any type of workflow requested by the client. We also provide a set of distinct flexible engagement models for our clients to select the most optimal solution for their business. We assist our customers to get the required edge that is needed to take their present business to next level by delivering various out-reaching technologies.
Get advanced technology that will help you find the
right answers for your every business need.
Get in touch
Drop us a line! We are here to answer your questions 24/7.