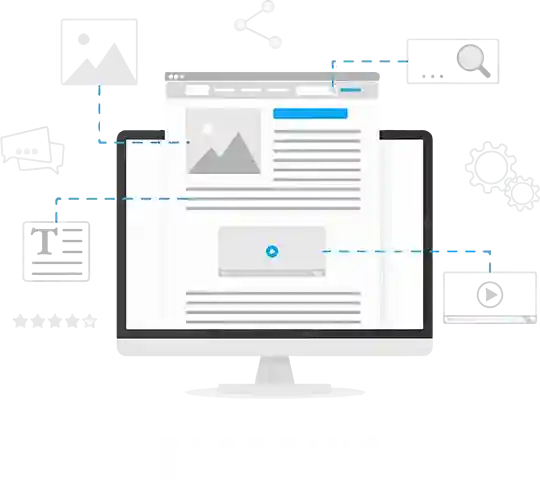
iFour Team - November 26, 2020
In Xamarin.Forms we run the code on the different mobile platforms and every platform has its own file system that is different from other platforms.All platforms have their own file system to read, write, and delete the file. In Xamarin.Forms there is no any direct API to use the local file system using Xamarin.Forms.
Using PCLStorage that is a third-party component, we can share the file storage library with all the platforms inside the shared code so there is no need to write code individually.PCLStorage provides a compatible and portable IO APIs of the local file system for .NET, and, Xamarin forms mobile platforms and for Silverlight. It is easy to develop cross-platform .Net applications and libraries.
To use the file storage library, we have to install this third-partycomponent PCLStorage from the NuGet package manager. For that right-click on the project solution. Click on the Manage NuGet package for the solution, search for PCLStorage, and install it. We are also able to install it from the package manager console. For that write "Install-Package PCLStorage -Version 1.0.2" into the console.
We can use System.IO classes to access the file system on each platform. It is used for creating, reading, and deleting the file. Directory class allows us to create and delete or enumerate the content of the directories. The stream subclass can be used to generate a degree of control on the file operation.
File.WriteAllText()method is used to write the file."File.WriteAllText(stfilename,text);"
File.ReadAllText() method is used to read the file. "File.ReadAllText(filename);"
To check if file is existing or not File.Exists() method can be used. "File.Exsists(fileName);"
We can get all the folders and paths of all the platforms with the help of PCLStorage. The below code (for cross-platform local folder) will help us to find it out. For this, we do not have to write code for any of platform to access the local folder.
Using PCLStorage; IFolder folder= FileSystem.Current.LocalStorage;
Here is code for creating a new folder inside local storage, we will call the CreateFolderAsync ()
String FolderName =”LocalFileSotage” ; IFolder folder = FileSystem.Current.LocalStorage; folder = await folder.CreateFolderAsync(FolderName, CreationCollisionOption.ReplaceExisting);
Code for creating new filewe will call CreateFileAsync ()
String FileName=”textfile.txt”; IFolder folder = FileSystem.Current.LocalStorage; IFile file = await folder.CreateFileAsync(FileName,CreationCollisionOption.ReplaceExisting);
Code for checking file if it is already existing or not we will use IsFileExistAsync ()
public async static TaskIsFileExistAsync(this string FileName, IFolderrootFolder = null) { IFolder folder = rootFolder ??FileSystem.Current.LocalStorage; ExistenceCheckResultfolderexist = await folder.CheckExistsAsync(fileName); if (folderexist == ExistenceCheckResult.FileExists) { return true; } return false; }
Code for checking folder if it is already existing or not we will use IsFolderExistAsync ()
public async static TaskIsFolderExistAsync(this string FolderName, IFolderrootFolder = null) { IFolder folder = rootFolder ??FileSystem.Current.LocalStorage; ExistenceCheckResultfolderexist = await folder.CheckExistsAsync(folderName); if (folderexist == ExistenceCheckResult.FolderExists) { return true; } return false; }
Code for writing content inside the file for that we will use WriteTextAllAsync ()
public async static TaskWriteTextAllAsync (this string Filename, string content = "", IFolderrootFolder = null) { IFile file = await filename.CreateFile(rootFolder); await file.WriteAllTextAsync(content); return true; }
Code for reading the file from the local storage we will use ReadAllTextAsync ()
public async static TaskReadAllTextAsync (this string FileName, IFolderrootFolder = null) { string content = " "; IFolder folder = rootFolder ??FileSystem.Current.LocalStorage; bool exist = await fileName.IsFileExistAsync(folder); if (exist == true) { IFile file = await folder.GetFileAsync (FileName); content = await file.ReadAllTextAsync(); } return content; }
Code for deleting file, we will use DeleteAsync ()
public async static TaskDeleteFile(this string FileName, IFolderrootFolder = null) { IFolder folder = rootFolder ??FileSystem.Current.LocalStorage; bool exist = await fileName.IsFileExistAsync(folder); if (exist == true) { IFile file = await folder.GetFileAsync(FileName); await file.DeleteAsync(); return true; } return false; }
Below code shows PCL Helper class using that class we can use local file storage.
using PCLStorage; using System; using System.Threading.Tasks; namespace LocalStorage { public static class PCLHelper { public async static TaskIsFileExistAsync (this string FileName, IFolderrootFolder = null) { IFolder folder = rootFolder ??FileSystem.Current.LocalStorage; ExistenceCheckResultfolderexist = await folder.CheckExistsAsync (FileName); if ( folderexist == ExistenceCheckResult.FileExists) { return true; } return false; } public async static TaskIsFolderExistAsync (this string FolderName, IFolderrootFolder = null) { IFolder folder = rootFolder ??FileSystem.Current.LocalStorage; ExistenceCheckResultfolderexist = await folder.CheckExistsAsync(folderName); if (folderexist == ExistenceCheckResult.FolderExists) { return true; } return false; } public async static TaskCreateFolder (this string folderName, IFolderrootFolder = null) { IFolder folder = rootFolder ??FileSystem.Current.LocalStorage; folder = await folder.CreateFolderAsync(folderName, CreationCollisionOption.ReplaceExisting); return folder; } public async static TaskCreateFile(this string FileName, IFolderrootFolder = null) { IFolder folder = rootFolder ??FileSystem.Current.LocalStorage; IFile file = await folder.CreateFileAsync(filename, CreationCollisionOption.ReplaceExisting); return file; } public async static TaskWriteTextAllAsync (this string FileName, string content = "", IFolderrootFolder = null) { IFile file = await filename.CreateFile(rootFolder); await file.WriteAllTextAsync(content); return true; } public async static TaskReadAllTextAsync (this string FileName, IFolderrootFolder = null) { string content = ""; IFolder folder = rootFolder ??FileSystem.Current.LocalStorage; bool exist = await FileName.IsFileExistAsync(folder); if (exist == true) { IFile file = await folder.GetFileAsync(FileName); content = await file.ReadAllTextAsync(); } return content; } public async static TaskDeleteFile(this string FileName, IFolderrootFolder = null) { IFolder folder = rootFolder ??FileSystem.Current.LocalStorage; bool exist = await fileName.IsFileExistAsync(folder); if (exist == true) { IFile file = await folder.GetFileAsync(fileName); await file.DeleteAsync(); return true; } return false; } } } }
Using the above code, we are able to read, write, create, and delete files and can check if files or folders already exist in local storage or not.
In this blog, we have understood how local file storage can work using Xamarin Forms.We have used PCLStorage competent to implement local file storage for a different platform of Xamarin forms. We have framed code for creating folder and file check if they exist or not and delete, write, and read the file, also created PCL Helper class.
January 23, 2023
September 21, 2021
September 20, 2021
July 23, 2021
July 06, 2021
Technology that meets your business requirements
Planning a cutting-edge technology software solution? Our team can assist you to handle any technology challenge. Our custom software development team has great expertise in most emerging technologies. At iFour, our major concern is towards driving acute flexibility in your custom software development. For the entire software development life-cycle, we implement any type of workflow requested by the client. We also provide a set of distinct flexible engagement models for our clients to select the most optimal solution for their business. We assist our customers to get the required edge that is needed to take their present business to next level by delivering various out-reaching technologies.
Get advanced technology that will help you find the
right answers for your every business need.
Get in touch
Drop us a line! We are here to answer your questions 24/7.